Running Guest OS Operations
Guest OS operations manipulate processes, files, folders, and environment variables in a virtual machine's guest operating system.
The vSphere API offers the following managed object types for guest operations:
- GuestAuthManager – authenticate to acquire credentials in the guest OS.
- GuestFileManager – manipulate files, directories, and remote copying in the guest OS.
- GuestProcessManager – manipulate processes in the guest OS.
- GuestAliasManager – support single sign-on for guest operations; create and delete user aliases.
- GuestWindowsRegistryManager – manipulate keys and values in the Windows registry.
- VirtualMachineGuestCustomizationManager – customize guest settings, especially for instant clone virtual machines.
All the above managed objects are subclasses that inherit properties from GuestOperationsManager. In the vSphere API, the VirtualMachine and GuestInfo managed objects contain information about what guest operations might be running and relevant virtual machine state:
vim.VirtualMachine.guest() vim.vm.GuestInfo.guestOperationsReady vim.vm.GuestInfo.interactiveGuestOperationsReady
- Java or C# program translates to SOAP bindings.
- Directives of vSphere API pass through vCenter Server.
- Directives are relayed to ESXi host agent process.
- Virtual machine executable passes guest operations to VMware tools.
- Guest OS performs guest operations.
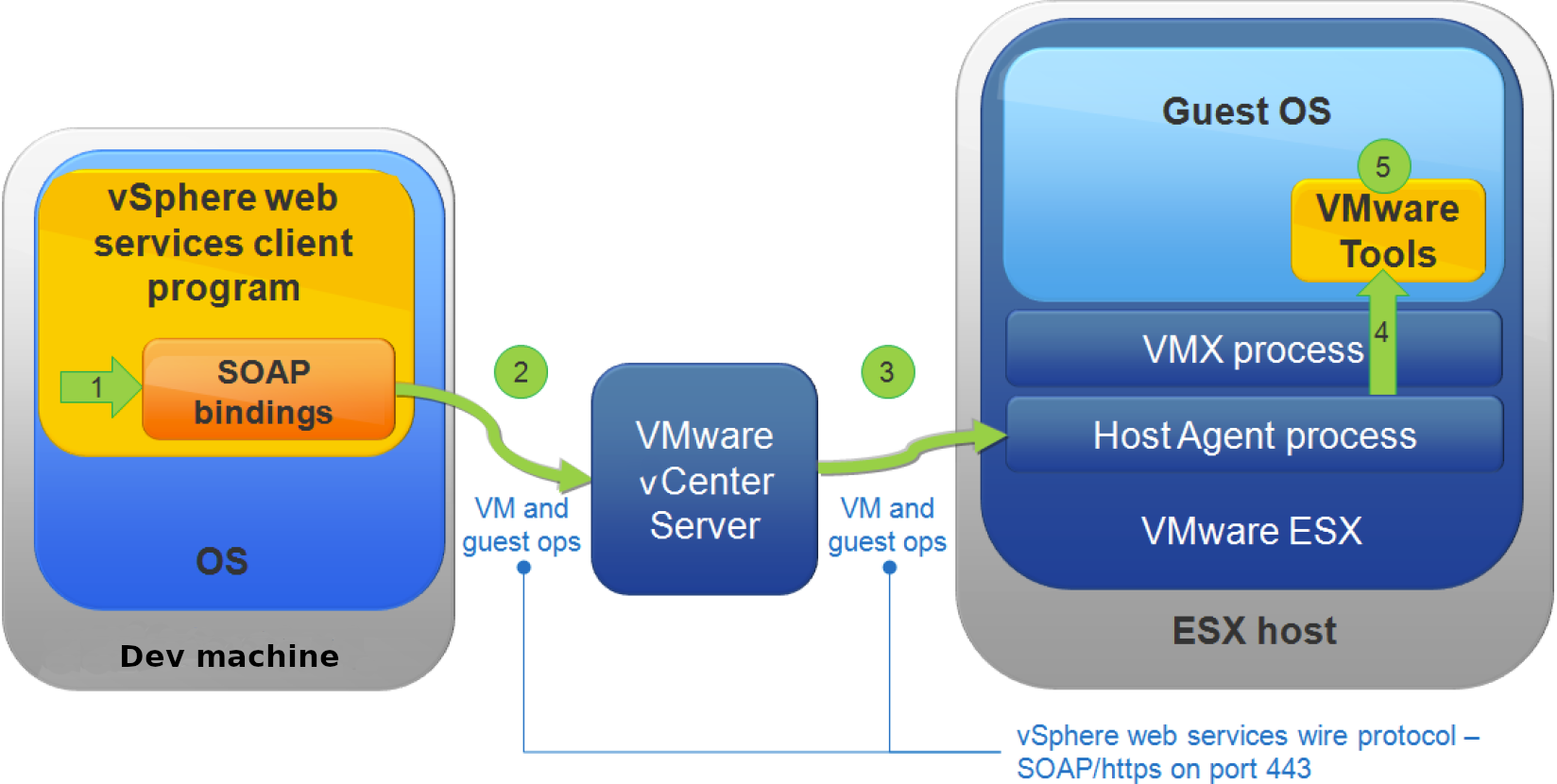
The following table summarizes the methods available for guest operations.
Managed Object | Methods | Description |
---|---|---|
GuestAliasManager | AddGuestAlias | define alias for guest account |
ListGuestAliases | list guest aliases for specified user | |
ListGuestMappedAliases | list alias map for in-guest user | |
RemoveGuestAliasByCert | remove certificate associated aliases | |
GuestAuthManager | AcquireCredentialsInGuest | authenticate, return session object |
ReleaseCredentialsInGuest | release session object | |
ValidateCredentialsInGuest | check authentication data or timeout | |
GuestFileManager | ChangeFileAttributesInGuest | change attributes of file in guest |
CreateTemporaryDirectoryInGuest | make a temporary directory | |
CreateTemporaryFileInGuest | create a temporary file | |
DeleteDirectoryInGuest | remote directory in guest OS | |
DeleteFileInGuest | remove file in guest OS | |
InitiateFileTransferFromGuest | start file transfer from guest OS | |
InitiateFileTransferToGuest | start file transfer to guest OS | |
ListFilesInGuest | list files or directories in guest | |
MakeDirectoryInGuest | make a directory in guest | |
MoveDirectoryInGuest | move or rename a directory in guest | |
MoveFileInGuest | rename a file in guest | |
GuestWindowsRegistryManager | CreateRegistryKeyInGuest | create a registry key |
DeleteRegistryKeyInGuest | delete a registry key | |
DeleteRegistryValueInGuest | delete a registry value | |
ListRegistryKeysInGuest | list registry subkeys for a given key | |
ListRegistryValuesInGuest | list registry values for a given key | |
SetRegistryValueInGuest | set or create a registry value | |
GuestProcessManager | ListProcessesInGuest | list processes running in guest OS |
ReadEnvironmentVariableInGuest | read environment variable in guest | |
StartProgramInGuest | start running program in guest | |
TerminateProcessInGuest | stop a running process in guest |
InitiateFileTransferFromGuest and InitiateFileTransferToGuest are useful for transferring small files between the host and guest. For large file transfers, virtual machines should be connected to the network because networking transfers are much faster.
Java Source Code Samples
Four Java code samples based on JAX-WS are available in the vSphere SDK for Web services, in this directory:
SDK/vsphere-ws/java/JAXWS/samples/com/vmware/guest
CreateTemporaryFile.java creates a temporary file inside a virtual machine, by calling the following method:
vimPort.createTemporaryFileInGuest(fileManagerRef, vmMOR, auth, prefix, suffix, directoryPath);
DownloadGuestFile.java downloads a file from the guest to
a specified path on the host where the client is running. The destination, a local
file on the client host, is specified on the command line as
--localfilepath
.
vimPort.initiateFileTransferFromGuest(fileManagerRef, vmMOR, auth, guestFilePath);
RunProgram.java runs
a specified program inside a guest operating system, with output re‐directed to a
temporary file, and downloads the resulting output to a file on the local client.
The program must already exist on the guest, and is specified on the command line as
--guestprogrampath
. The output file to store on the client host
is specified on the command line as --localoutputfilepath
.
vimPort.startProgramInGuest(processManagerRef, vmMOR, auth, spec);
UploadGuestFile.java
uploads a file from the client machine to a specified location inside the guest. The
source, a local file on the client host, is specified on the command line as
--localfilepath
.
vimPort.initiateFileTransferToGuest(fileManagerRef, vmMOR, auth, guestFilePath, guestFileAttributes, fileSize, optionsmap.containsKey("overwrite"));